We gone through how the connection between Raspberry Pi and DC motors works in Part I. And now, it's time to learn how to give control signals from web application to Raspberry Pi and control DC motors.
Pre-requisites
Raspberry Pi with Raspbian OS installed
Install Flask using pip as follows.
What is RPi.GPIO package?
It provides a class to control the GPIO of Raspberry Pi. This package is not suitable for real-time applications because of Python will be busy in garbage collection which cannot be predicted and it's multitasking OS and another process can be given higher priority over CPU causing jitter in the program.
Steps to Code:
The folder structure of our project is as follows.
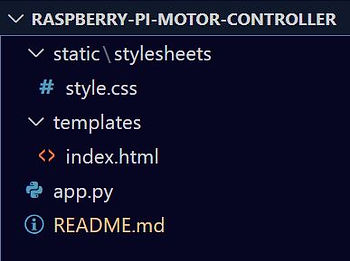
Step 1: Create file index.html inside templates folder
Flask will look for templates in the template folder. So, create the file index.html inside template folder of the main module. Use string interpolation {{ variable_name }} to print the motor input status which will be provided in keyword arguments.
In User Interface (UI) shown in Fig 1, we have 5 action buttons - Straight, Reverse, Left, Right and Stop. While clicking on STRAIGHT button, the '/straight' hyperlink will be called. In the same way, for each button the action path variable will be changed to move the wheels in desired direction.
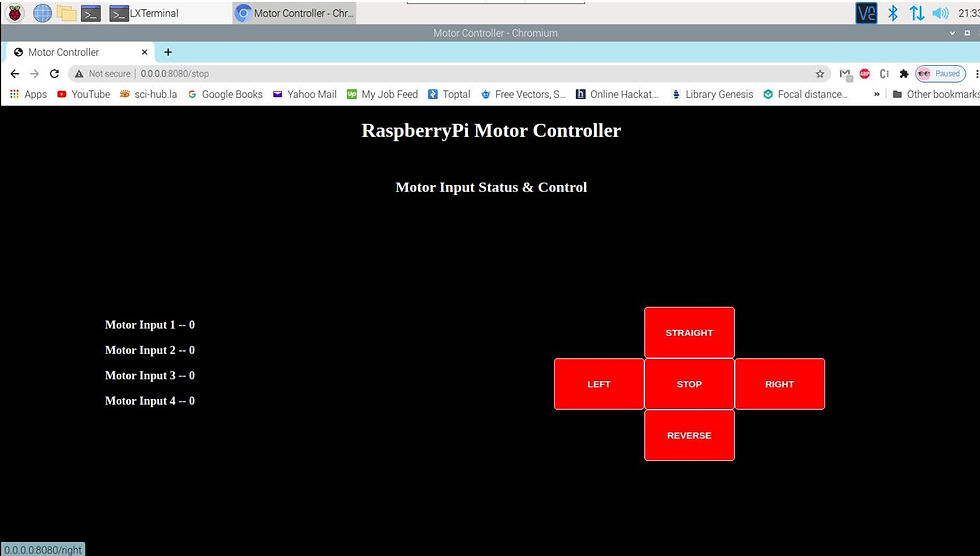
Step 2: Create file app.py
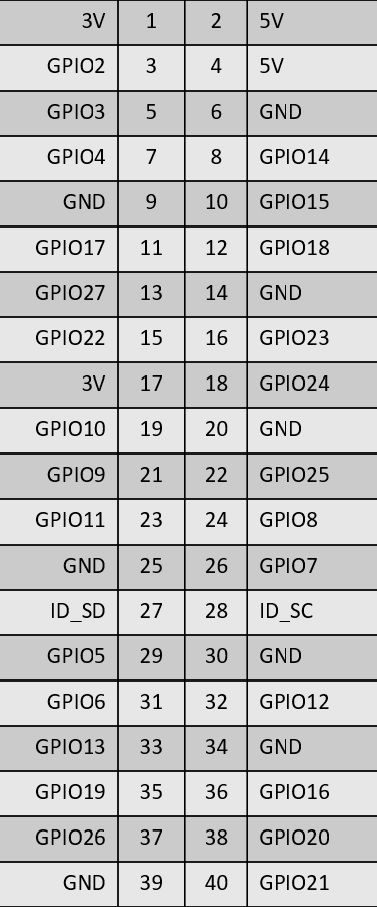
Create a python file app.py in the main module.
Import the library RPi.GPIO and set the pin mode. There are two types of GPIO mode. GPIO.BOARD which uses pin numbers and GPIO.BCM which uses GPIO numbers. Refer the pin configuration figure. The center two rows are pin numbers and the outer rows (1st and 4th) are GPIO numbers. In our code, we are setting the pin mode to GPIO.BCM as below.
Now define the GPIO pins 18, 23, 24 and 35 as output.
GPIO.input() is used to read GPIO status and GPIO.output() is used to write GPIO status.
The render_template() method is used to render a template. The name of the template and the variables that has to be passed to the Jinja2 template engine as keyword arguments will be provided in this method. When the '/' api is called on load, all the motor input status will be read and passed to the template.
For each actions passed in the path variable from user interface, the GPIO output will be set accordingly using the below code. For example, when straight action is triggered, set the variables motorIn1 and motorIn3 to GPIO.HIGH and motorIn2 and motorIn4 to GPIO.LOW which will lead to forward motion of the RC car ( Detailed explanation is given in Part I ).
The motor input status of GPIO pins for straight action will be as in below screenshot.
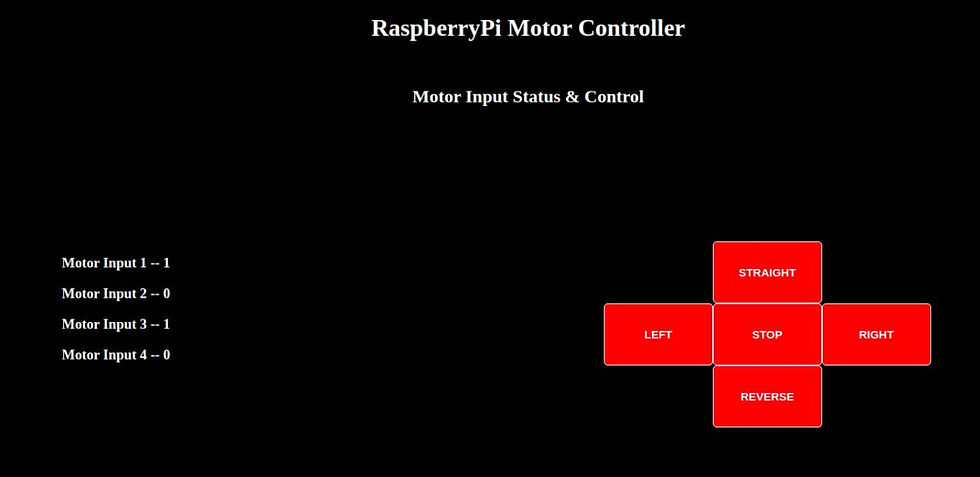
Step 3: Run app.py
Run the app.py using the below command.
After the application runs successfully, open the application in default port 8080. The index.html template will be rendered on load along with motor input status.
Now, we are all set to controll our RC car using the Motor Controller UI. Happy driving!!
Note: The full source code is available in GitHub.
Comments